How to create and run java Program
Table of Contents
Java is a general-purpose, object-oriented programming language. Java consists of a programming language, a compiler, core libraries and a runtime also called Java virtual machine. The Java platform is usually associated to two core components the Java virtual machine and the Java core libraries. To create a Java program, you need an editor and to run a java program java runtime environment is needed.
Java Licensing
As Java project is managed by oracle so Java Development Kit can be downloaded from Java Website or oracle website . Open JDK has GPL and it is free for personal use and developers. Commercial License and Support is available with a low cost subscription.
Java Platform offers two types of Application Development Editions
- Java Standard Edition (Java SE)
- Java Enterprise Edition (Java EE)
Java Standard Edition (Java SE)
Java SE offers developers to write applets and java applications using Java Technology. Java SE contains Java Development Kit.
Java Development kit
Java Development Kit is a bundle of software used to develop Java Applications or applets using java programming Language. For Developers Java Development Kit is needed, Java8 can be downloaded from Java SE.
Java Runtime Environment
Java Runtime environment is a very small program which enables the Java applets or applications to run. JRE implements Java Virtual Machine (JVM) in order the execute Java applications.
JRE can be downloaded independently from Java website , and it is also integrated in JDK as well.
In Java SE, programs can be developed.
- Stand-alone applications
- Web Applets
Stand-alone applications
Stand-alone applications are programs written in Java to carry out certain tasks on a stand-alone local computer. A stand-alone Java program can be executed in two steps:
- Compiling source code into byte code using Java compiler(javac.exe).
- Executing the byte code program using Java interpreter(java.exe).
Web Applets
Java Applet is a very small program that is embedded in the webpage to generate the dynamic content. It runs inside the browser as a small window on client machine. It is in particular helpful in getting user feedbacks. You simply need a java compatible browser to run an applets.
Java Enterprise Edition
Java Enterprise Edition as name suggest focuses on the applications for enterprise environment. It offers web centric online solutions which are platform-independent for enterprises. Java EE includes many components of the Java Standard Edition (Java SE).The Java EE platform consists of a set of services, APIs, and protocols that provide the functionality for developing multitiered, Web-based applications.
Java EE greatly simplifies application development process by putting in place reusable modular components to handle multitier capabilities of Java EE automatically. These reusable components reduces programming code by significant margin and hence saving a lots of time and efforts.
Distributed business applications are not so easy to develop, and they need a high-productivity solution that allows them to focus only on writing their business logic and having a full range of enterprise-class services to rely on, like transactional distributed objects, message oriented middleware, and naming and directory services.
Java EE can be downloaded from Java EE.
As already mentioned, JavaSE or JavaEE can be downloaded from java.com. Other versions of Java are available for linux, solaris and mac os x.
Download the version as per your operating system on x86 or x64 bits platform.
Installing Java
Double click the file downloaded from java website. Installation will show welcome screen and if you wish to change the destination folder, select the checkbox and hit install. Change the destination folder and click next to complete installation of Java.
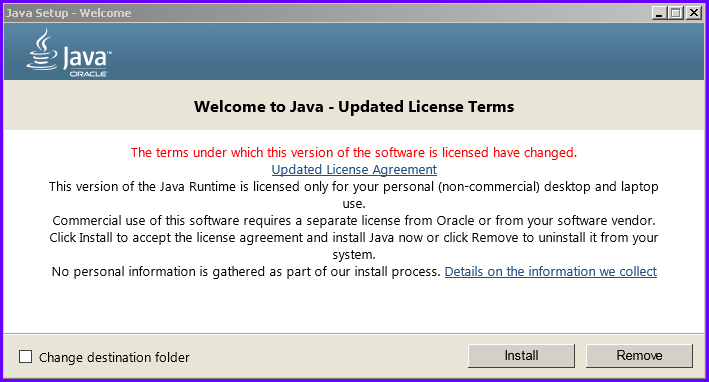
Setting Class path for Java
Java 7,8 configures its class path automatically. But the previous versions required the path to be set manually. Javac and java files resides in the bin folder of java. To set class path manually follow the steps as under:
1 Right click my Computer >select Properties
2 Under Advanced tab > select Advanced System Settings
3 Under System variables > Click on Environment variables
4 Select path and click edit (Caution: don’t delete it).
5 In the variables values textbox, scroll to end, put semi colon before pasting the java program files path (Java path can be copied as per your installation directory). Hit ok three times to save path.
Configure Java
To configure Java, Java Control Panel is used. It is available in all programs > java > configure java. Java Control Panel contains five tabs namely, general, update, java, security and advanced.
General tab shows the version of java, network settings and temporary internet files.
You can disable automatic update of java by removing the check box in update tab.
Java tab is used to manage multiple java versions.
Security tab configures security and exception lists.
More advanced features such as SSL, TLS, console, debugging etc. are configured on advanced tab.
Integrated Development Environment for Java
Like HTML, C/C++ Java also need an editor to write and edit codes. In case you don’t have one, then you can simply use notepad. and compile and run the java programs from command prompt.
This system needs you to remember the commands and you need to set the class path if it is not set automatically during installation.
Writing a Simple Java Program
Let us write a simple Java program in notepad.
// A Simple Java Program. class MyPrg { public static void main(String args[]) { System.out.println(“A simple Java Program”); } }
Naming a Java File
Once you complete typing this program in notepad, save the file in a folder, file name must match the name of class (preserving the case) with extension “.java” e.g. save it as “MyPrg.java”.
Compile a Java Program
Now go to command prompt. Change the directory to the path where java code file has been saved.
To compile a java program type “javac MyPrg.java” and hit enter key.
Again cursor will get back to command prompt without any message if compilation is successful. otherwise it will return some error.
A class file has been created by the compiler by the name “MyPrg.class”. This is bytecode which are machine independent and needs to be interpreted by the appropriate interpreter.
To Interpret/run Java Program
To interpret the class file, type the command as under:
“java MyPrg” and hit enter key on the command prompt.
It will show the result.
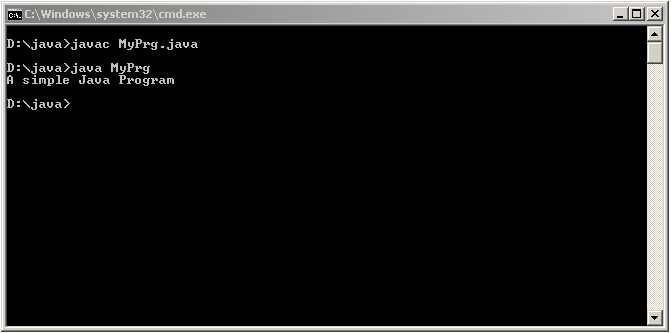
Writing a Java Program using Eclipse Editor
Another option is to use specially designed IDE, such as Eclipse. Eclipse is very famous IDE used for java, C/C++, PHP and many more languages. It has JRE inbuilt so that programs can be directly compiled and run from within the same IDE. Eclipse can be downloaded from eclipse.com
Writing and Compiling Java Program using Eclipse Editor
- Open eclipse editor and click on file > new > Project
- Type the name of the project , select the JRE environment version to execute the java project and click finish.
- Now right click on the src folder on the left side of project window. Click new > select class
Run Java Program - Type the name of the class
- From which method stub would you like to create, Select the checkbox “public static void main(String[] args)” and click finish.
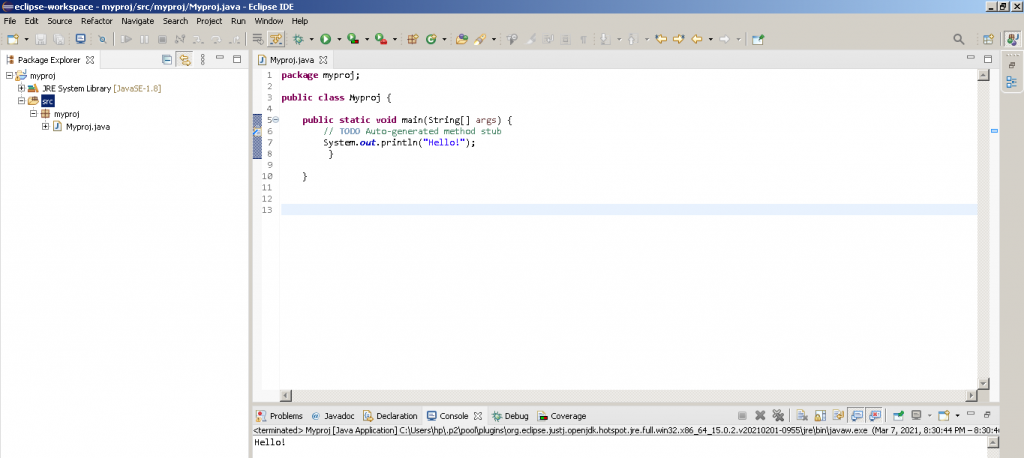
Once you finish typing java codes, Simply click on run icon or Control+F11 keys from keyboard.
Comments in Java
The main utility of comments is that it passes the message to the fellow programmer about the logic used but the same is ignored by the compiler during compilation.
Comments are of two types-
- Single Line Comments
Two forward slashes in the beginning of a statement are called single line comments. eg. // A Hello Java Program.
- Multiline Comments
These comments begin with /* and ends with */. It can span multiple lines in one go.
Class Declaration
You must be declared in a class, using keyword Class e.g, class MyPrg. here is used to declare a class. A class is a logically grouping of objects. The objects contains data and the methods.
Open Brace
In Java, every class is defined between opening braces ”{“ and closing braces “}“ . Pair of braces defines the scope of any method or class.
The Main method
public static void main(String args[])
or it can also be written as :
public static void main(String[] args)
The main() method is the beginning of the program. All Java applications starts execution by calling main(). The main() must declared as public, because it should also be called by the code outside of its class when the program is started.
The keyword void simply tell the compiler that main() does not return a value.
The keyword static allows main() to be called without having instantiate a particular instance of the class.
In main(), there is only one parameter, String args[] which declares a parameter named args, which is an array of instances of the class string.
The Output Line
The next line of code is shown here:
System.out.println(“Hello”);
This line output the string “Hello” , followed by a new line on the screen. Output is accomplish by the built-in println() method.
System is a predefined class that provides access to the system, and out is the output stream that is connected to the console.
All statements in Java end with a semicolon (;).
Another Program in Java
Let us write a program to calculate the square root of a number.
import java.math; class squareRoot { public static void main(String args[]) { double a = 5; // declaration and initialization double double sq; sq = math.sqrt(a); System.out.println(“sq =”+sq); } }
The structure of the program is similar to the previous one except that it has more number of statements in its main() function. The statement,
double a=5;
Declares a variable ‘a’ and initializes it to the value 5.
The statement
double sq;
Declares a variable ’sq’.
Both the variables ‘a’ and ‘sq’ are declared as double type as to represent a floating point fractional number.
The statement
Sq = Math.sqrt(a);
Invokes the method sqrt available in Math class, computes the square root of ‘a’ and assign the result to the variable ‘sq’.
The output statement
System.out.println(“sq=”+sq);
Displays the result on screen as
Sq = 2.23607
‘+’ symbol acts as the concatenation operator of two strings.
Use of Math Functions
Consider the first statement of the program:
import java.math;
This statement instructs the interpreter of Java to load the math class from the package java. This Math class contains the sqrt method used in the above program.
An Application with Two Classes
The two examples discussed above contain only one class that contains main () method. Multiple classes are required in a real-life application. Let’s consider a program in Java with two classes.
//program with two classes class Box { double width; double height; double length; } // This class declares an object of type Box. class BoxDemo { public static void main(String args[]) { Box mybox = new Box() { Box mybox = new Box(); double vol; //assign values to mybox’s instance variables Mybox.width =10; Mybox.height = 15; Mybox. Length = 20; // computer volume of the box vol = mybox.width * mybox.height *mybox.length; System.out.println (“volume is” +vol); } }
The above program contains two classes Box and BoxDemo. The Box class defines three variables. The class BoxDemo contains the main method that initiates the execution.
The main method create a Box object by the statement,
Box mybox = new Box();
Tells the compile to assign the copy of width that is contained within the mybox object the value equal to 10.
A local variable vol is declared which stores the volume of the object mybox. The volume of the box is given as output of the above program. The output produced by the above program is:
Volume is 300.0
Conclusions: Java is very secure, robust, modular and object oriented Programming Language. I have explained about how to run a java program from beginners point of view . Please like, share and comment.
Similar Topics: Features of Java Object Oriented Programming Features
You May Like it: Learning Account Groups in Tally Prime C Language Introduction Where to write JavaScript Code
[…] May Also Like: How to create and run java Program Elements of C Language […]